Store Data in to Micro-Services Static class using c# dot net core (Key Based API for Reduce Database hit)
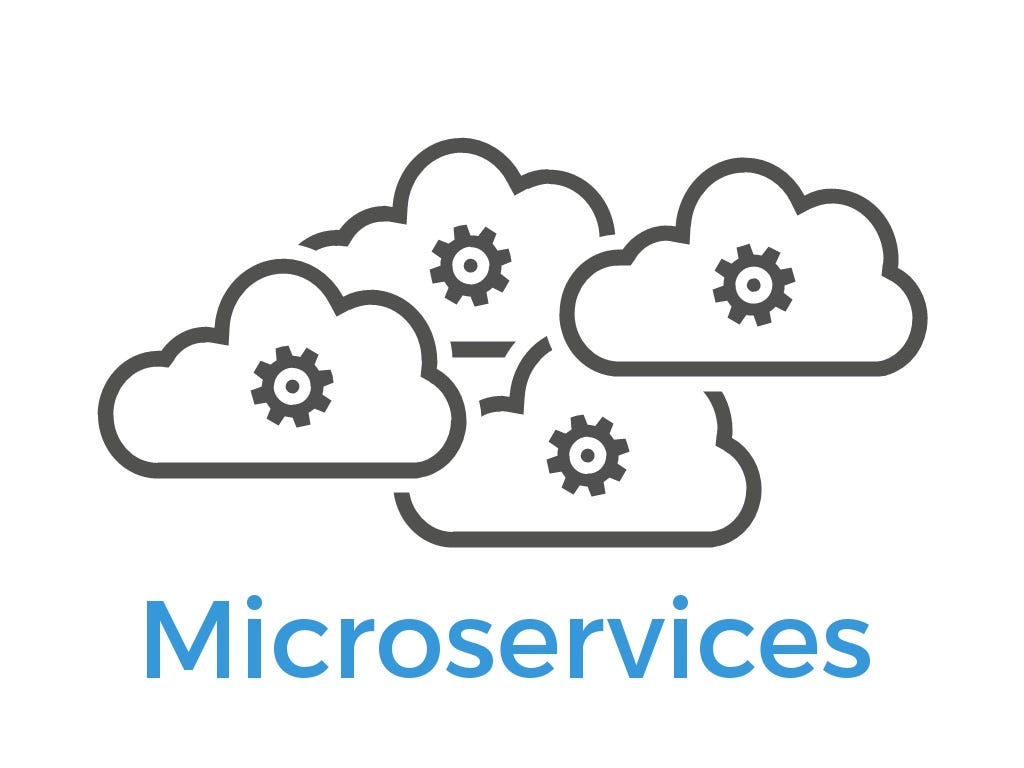
Find Code From Here : Code
Testing : 1. 1st Time Entry with Null key value and Adding New Value into Class
Testing : 2 . 2nd Time Entry with unique key value and updating existing entry into Class
Testing : 3. Same Time Another Hit Came From different User for for update Data
Testing 4: If Step is 4 then we are inserting data into our database and Deleting data from class
using System;
using System.Collections.Generic;
using System.Data;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
// For more information on enabling Web API for empty projects, visit https://go.microsoft.com/fwlink/?LinkID=397860
namespace AppForStaticCls.Controllers
{
[Route("api/[controller]")]
public class MutiplePostController : Controller
{
// GET: api/<controller>
[HttpGet]
public IEnumerable<string> Get()
{
return new string[] { "value1", "value2" };
}
// POST api/<controller>
[HttpPost]
public List<TestDataRes> Post(string key, string step, string name, string address, string country)
{
string newKey = "";
List<TestDataRes> testDataResList = new List<TestDataRes>();
if (TestData.count == 0)
{
newKey = Guid.NewGuid().ToString();
DataColumn nme = new DataColumn("name");
DataColumn adrs = new DataColumn("address");
DataColumn cntry = new DataColumn("country");
DataColumn createdDate = new DataColumn("dateTime");
DataColumn dtKey = new DataColumn("key");
TestData.Dt = new DataTable();
TestData.Dt.Columns.Add(nme);
TestData.Dt.Columns.Add(adrs);
TestData.Dt.Columns.Add(cntry);
TestData.Dt.Columns.Add(createdDate);
TestData.Dt.Columns.Add(dtKey);
TestData.count = TestData.count + 1;
DataRow row = TestData.Dt.NewRow();
row["key"] = newKey;
row["dateTime"] = DateTime.Now;
TestData.Dt.Rows.Add(row);
UpdateData(newKey, name, address, country);
}
else
{
if (!UpdateData(key, name, address, country))
{
newKey = Guid.NewGuid().ToString();
DataRow row = TestData.Dt.NewRow();
row["dateTime"] = DateTime.Now;
row["key"] = newKey;
TestData.Dt.Rows.Add(row);
UpdateData(newKey, name, address, country);
}
}
if (step == "4")
{
//will call database
IEnumerable<DataRow> rows = TestData.Dt.Rows.Cast<DataRow>().Where(r => r["key"].ToString() == key);
rows.ToList().ForEach(r => r.Delete());
}
if (key != null)
{
testDataResList = AddData(key);
}
else
{
testDataResList = AddData(newKey);
}
Flush();
return testDataResList;
}
public bool Flush()
{
IEnumerable<DataRow> rows = TestData.Dt.Rows.Cast<DataRow>().Where(r => Convert.ToDateTime(r["dateTime"]) <= DateTime.Now.AddMinutes(-30));
rows.ToList().ForEach(r => r.Delete());
return true;
}
public List<TestDataRes> AddData(string key)
{
List<TestDataRes> testDataResList = new List<TestDataRes>();
foreach (DataRow item in TestData.Dt.Rows.Cast<DataRow>().Where(r => r["key"].ToString() == key))
{
testDataResList.Add(new TestDataRes { name = item["name"].ToString(), address = item["address"].ToString(), country = item["country"].ToString(), key = key });
}
return testDataResList;
}
public bool UpdateData(string Key, string name, string address, string country)
{
if (!string.IsNullOrEmpty(Key))
{
IEnumerable<DataRow> rows = TestData.Dt.Rows.Cast<DataRow>().Where(r => r["key"].ToString() == Key);
if (!string.IsNullOrEmpty(name))
{
rows.ToList().ForEach(r => r.SetField("name", name));
}
if (!string.IsNullOrEmpty(address))
{
rows.ToList().ForEach(r => r.SetField("address", address));
}
if (!string.IsNullOrEmpty(country))
{
rows.ToList().ForEach(r => r.SetField("country", country));
}
return true;
}
else
{
return false;
}
}
}
public static class TestData
{
public static DataTable Dt { get; set; }
public static int count { get; set; }
}
public class TestDataRes
{
public string name { get; set; }
public string address { get; set; }
public string country { get; set; }
public string key { get; set; }
}
}
Store Data in to Micro-Services Static class using c# dot net core (Key Based API for Reduce Database hit)
Reviewed by Ashwani
on
March 25, 2020
Rating:

No comments:
Post a Comment